Label images with a deep neural network
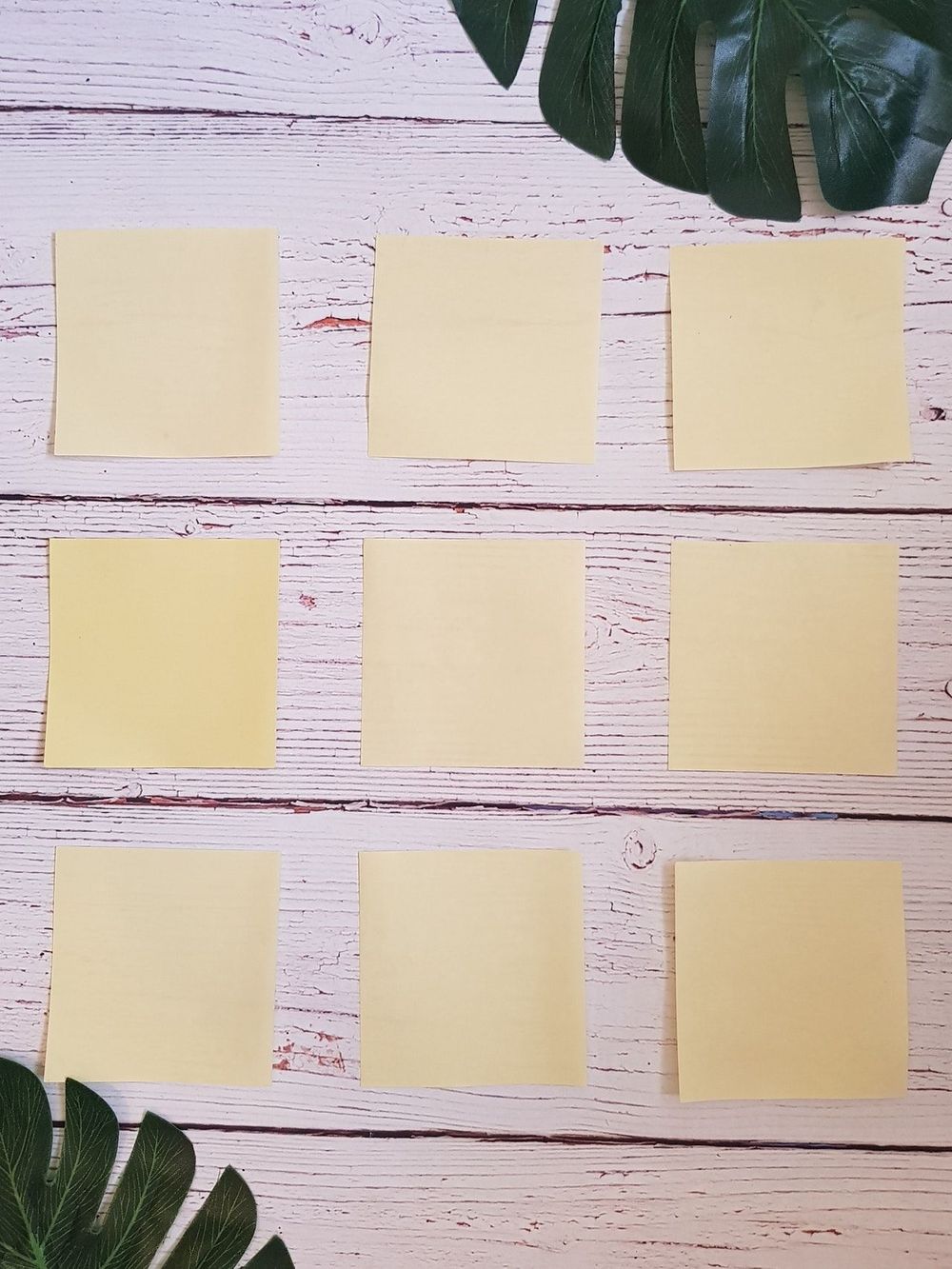
It isn't often that you need to label lots of images. But when the need arises you can use many of the pre-trained deep neural network models available for image labeling tasks. One of the most popular ones is ResNet50 and keras
provides a convenient of using it.
SETUP
I'm a big fan of docker
and will use gw000/keras docker
image that comes installed with the necessary libraries needed to use ResNet50 for image labeling. A few additional tweaks are still needed and I'll build a custom docker
image on top of gw000/keras.
First, create a directory in your workspace and cd
into it.
mkdir resnet50labeling
cd resnet50labeling
Create a Dockerfile.
touch Dockerfile
Paste the following into your Dockerfile.
FROM gw000/keras:2.1.4-py2-tf-cpu
# install dependencies from debian packages
RUN apt-get update -qq \
&& apt-get install --no-install-recommends -y \
python-matplotlib \
python-pillow \
wget
WORKDIR /
Build the image.
docker build -t resnet50labeling:v1 .
Create container from the image and shell into it.
docker run -it resnet50labeling:v1 /bin/bash
LABELING IMAGES
Once inside the container download an image to label. The command below downloads an image of a golden retriever.
wget https://i.ytimg.com/vi/SfLV8hD7zX4/maxresdefault.jpg
Next, start a Python session inside the container and get ready to label the image downloaded above. The Python snippet below will be used to label the downloaded image. While executing the snippet below, a large file download will take place. This file contains the pre-trained weights of the ResNet50 deep neural network that is necessary to label the image.
import keras
from keras.applications.resnet50 import ResNet50
from keras.preprocessing import image
from keras.applications.resnet50 import preprocess_input, decode_predictions
import numpy as np
model = ResNet50()
input_img_path = 'maxresdefault.jpg'
img = image.load_img(input_img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
predictions = model.predict(x)
print('Predicted:', decode_predictions(predictions, top=3)[0])
If all goes well you should see the following;

REFERENCES
ResNet50 is a large deep neural network architecture trained on more than 1 million images on ImageNet. This paper and this has more details on the original ResNet models.
ResNet50 in Keras defines the deep neural network architecture and provides a convenient way to use it as I've shown in this post.